Commit bed9952
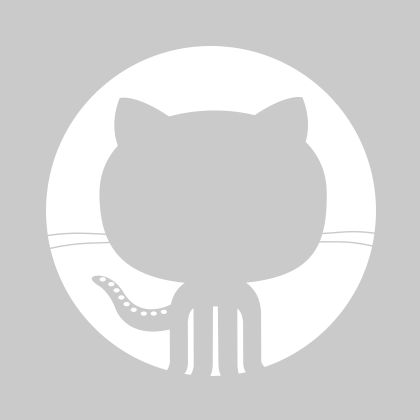
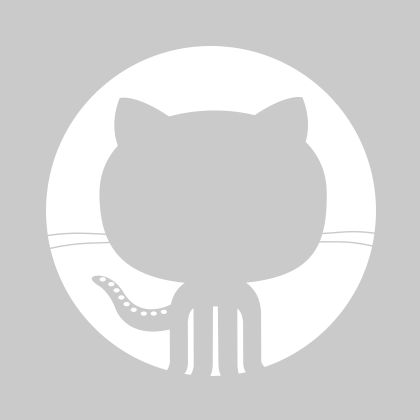
Patil, Omkar
Patil, Omkar
1 parent 52f50d2 commit bed9952
File tree
11 files changed
+259
-137
lines changed- generators/app
- templates
- src
- typings
- test
11 files changed
+259
-137
lines changed+56-13
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
4 | 4 |
| |
5 | 5 |
| |
6 | 6 |
| |
7 |
| - | |
8 |
| - | |
9 |
| - | |
10 |
| - | |
11 |
| - | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
12 | 13 |
| |
13 | 14 |
| |
14 | 15 |
| |
15 | 16 |
| |
16 | 17 |
| |
17 |
| - | |
| 18 | + | |
18 | 19 |
| |
19 | 20 |
| |
20 |
| - | |
| 21 | + | |
21 | 22 |
| |
22 | 23 |
| |
23 |
| - | |
24 |
| - | |
25 |
| - | |
26 |
| - | |
| 24 | + | |
27 | 25 |
| |
28 | 26 |
| |
29 | 27 |
| |
| |||
36 | 34 |
| |
37 | 35 |
| |
38 | 36 |
| |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
39 | 48 |
| |
40 |
| - | |
| 49 | + | |
41 | 50 |
| |
42 |
| - | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
| 69 | + | |
| 70 | + | |
| 71 | + | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
| 83 | + | |
| 84 | + | |
| 85 | + | |
43 | 86 |
| |
44 | 87 |
| |
45 | 88 |
| |
|
+27-18
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
4 |
| - | |
5 |
| - | |
6 |
| - | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
7 | 7 |
| |
8 | 8 |
| |
9 | 9 |
| |
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
15 |
| - | |
| 15 | + | |
16 | 16 |
| |
17 | 17 |
| |
18 | 18 |
| |
19 | 19 |
| |
20 |
| - | |
| 20 | + | |
21 | 21 |
| |
22 | 22 |
| |
23 |
| - | |
| 23 | + | |
24 | 24 |
| |
25 | 25 |
| |
26 | 26 |
| |
| |||
30 | 30 |
| |
31 | 31 |
| |
32 | 32 |
| |
| 33 | + | |
33 | 34 |
| |
34 | 35 |
| |
35 | 36 |
| |
36 | 37 |
| |
37 | 38 |
| |
38 | 39 |
| |
39 |
| - | |
40 |
| - | |
| 40 | + | |
| 41 | + | |
41 | 42 |
| |
42 | 43 |
| |
43 | 44 |
| |
44 | 45 |
| |
45 | 46 |
| |
46 | 47 |
| |
47 |
| - | |
| 48 | + | |
48 | 49 |
| |
49 | 50 |
| |
50 | 51 |
| |
51 |
| - | |
| 52 | + | |
52 | 53 |
| |
53 | 54 |
| |
54 | 55 |
| |
55 |
| - | |
| 56 | + | |
56 | 57 |
| |
57 | 58 |
| |
58 | 59 |
| |
59 |
| - | |
| 60 | + | |
60 | 61 |
| |
61 | 62 |
| |
62 | 63 |
| |
63 |
| - | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
64 | 69 |
| |
65 | 70 |
| |
66 | 71 |
| |
67 |
| - | |
68 |
| - | |
69 |
| - | |
70 |
| - | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
71 | 80 |
| |
72 | 81 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
2 |
| - | |
3 |
| - | |
| 1 | + | |
| 2 | + | |
| 3 | + | |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 |
| - | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
8 | 11 |
| |
9 |
| - | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
10 | 17 |
| |
11 | 18 |
| |
12 | 19 |
| |
13 |
| - | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
| 41 | + | |
| 42 | + | |
14 | 43 |
| |
15 |
| - | |
16 |
| - | |
| 44 | + | |
| 45 | + | |
17 | 46 |
| |
18 | 47 |
| |
19 |
| - | |
| 48 | + | |
20 | 49 |
| |
21 | 50 |
| |
22 | 51 |
| |
23 | 52 |
| |
24 | 53 |
| |
25 | 54 |
| |
26 | 55 |
| |
27 |
| - | |
28 |
| - | |
29 |
| - | |
30 |
| - | |
| 56 | + | |
| 57 | + | |
31 | 58 |
| |
32 |
| - | |
33 |
| - | |
34 |
| - | |
35 |
| - | |
36 |
| - | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
37 | 62 |
| |
38 |
| - | |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
16 | 16 |
| |
17 | 17 |
| |
18 | 18 |
| |
| 19 | + | |
19 | 20 |
| |
20 |
| - | |
21 | 21 |
| |
22 |
| - | |
| 22 | + | |
23 | 23 |
| |
| 24 | + | |
24 | 25 |
| |
25 |
| - | |
26 |
| - | |
| 26 | + | |
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
30 |
| - | |
| 30 | + | |
31 | 31 |
| |
32 | 32 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
3 |
| - | |
4 |
| - | |
5 |
| - | |
6 |
| - | |
7 |
| - | |
8 |
| - | |
9 |
| - | |
10 |
| - | |
11 |
| - | |
12 |
| - | |
13 |
| - | |
14 |
| - | |
15 |
| - | |
16 |
| - | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
17 | 17 |
|
0 commit comments