|
| 1 | +# Figma to Angular Converter |
| 2 | + |
| 3 | +This demonstrates using the Figma REST API to convert a Figma document to Angular Components and Figma components module. |
| 4 | +Disclaimer: this code is likely incomplete, and may have bugs. It is not intended to be used |
| 5 | +in production. This is simply a proof of concept to show what possibilities exist. |
| 6 | + |
| 7 | +## API Usage |
| 8 | + |
| 9 | +We use 2 endpoints in this project: |
| 10 | + |
| 11 | +- `GET /v1/files/:file_key` - Get the JSON tree from a file. This is the main workhorse of this project and lays the skeleton of the React Components. |
| 12 | +- `GET /v1/images/:file_key` - When we identify nodes that are vectors or other nodes that can't directly be represented by `div`s, we have to render them as svgs. |
| 13 | + |
| 14 | +## Set up |
| 15 | + |
| 16 | +1. Install [Node](https://nodejs.org/). You'll need a recent version that supports `async / await` |
| 17 | +2. In this directory, run `npm install` |
| 18 | +3. Run the converter per instructions below |
| 19 | + |
| 20 | +## Usage |
| 21 | + |
| 22 | +By default this project comes with a prerendered component in `src/figmaComponents`. You can see a page that uses this component if you |
| 23 | +run `npm start`. This will start a React server and a webpage will open to the root page. This webpage will automatically refresh as |
| 24 | +you make changes to the source documents. To follow along with the example component, the source Figma file is located [here](https://www.figma.com/file/VGULlnz44R0Ooe4FZKDxlhh4/Untitled). |
| 25 | + |
| 26 | +When we run the converter, we will convert any *top level frames* in the document to Angular Components *as long as their name starts with `#`*. |
| 27 | +In the example document you can see that we have one top level frame named `#Clock`. The component resulting from this will be exported in |
| 28 | +`src/components/CFClock.component` as `CFClock`, a `dump component`. |
| 29 | + |
| 30 | +In addition, *any* node with a name starting with a `#` will have a code stub generated for it in `src/components`. These code stubs can be |
| 31 | +modified to affect the rendering of those components as well as modifying variables within the component (see variables section below). |
| 32 | + |
| 33 | +To run the converter on a file, you will need a personal access token from Figma. Refer to the [Figma API documentation](https://www.figma.com/developers/docs) |
| 34 | +for more information on how to obtain a token. The other piece of information you will need is the file key of the file you wish to convert, |
| 35 | +which is located in the file's URL (for example, this is `VGULlnz44R0Ooe4FZKDxlhh4` for the example file). So, an example conversion would look |
| 36 | +like: |
| 37 | + |
| 38 | +``` |
| 39 | +node main.js VGULlnz44R0Ooe4FZKDxlhh4 <API_TOKEN_HERE> |
| 40 | +``` |
| 41 | + |
| 42 | +where `<API_TOKEN_HERE>` would be repaced with your developer token. |
| 43 | + |
| 44 | +You can also change the .env file and insert your token there |
| 45 | + |
| 46 | +``` |
| 47 | +//.env |
| 48 | +DEV_TOKEN=<YOUR_TOKEN> |
| 49 | +``` |
| 50 | +then you only need to pass the project id |
| 51 | +``` |
| 52 | +node main.js VGULlnz44R0Ooe4FZKDxlhh4 |
| 53 | +``` |
| 54 | + |
| 55 | + |
| 56 | + |
| 57 | +*IMPORTANT*: The index.css file is required to be included for components to render completely. |
| 58 | + |
| 59 | +## Variables |
| 60 | + |
| 61 | +The real vision of this converter is to separate design concerns from coding concerns. Toward this end, we introduce the concept of |
| 62 | +`variables` in Figma. Variables in a Figma file are denoted by text nodes (this can be expanded in the future) with names starting with |
| 63 | +`$`. In the example document there are three variables: `$time`, `$seconds`, and `$ampm`. By setting state in the component stubs defined in |
| 64 | +`src/components`, we can *change the text of the variable nodes*. For an example, take a look at `src/app/app.component`. This |
| 65 | +allows us to change the design of a component without touching the code at all. |
| 66 | + |
| 67 | +## Why Angular? |
| 68 | + |
| 69 | +Because it's one of the best frameworks |
| 70 | + |
| 71 | +## Examples |
| 72 | + |
| 73 | +Here's an example of using Figma to React to update the style on a sortable list: |
| 74 | + |
| 75 | +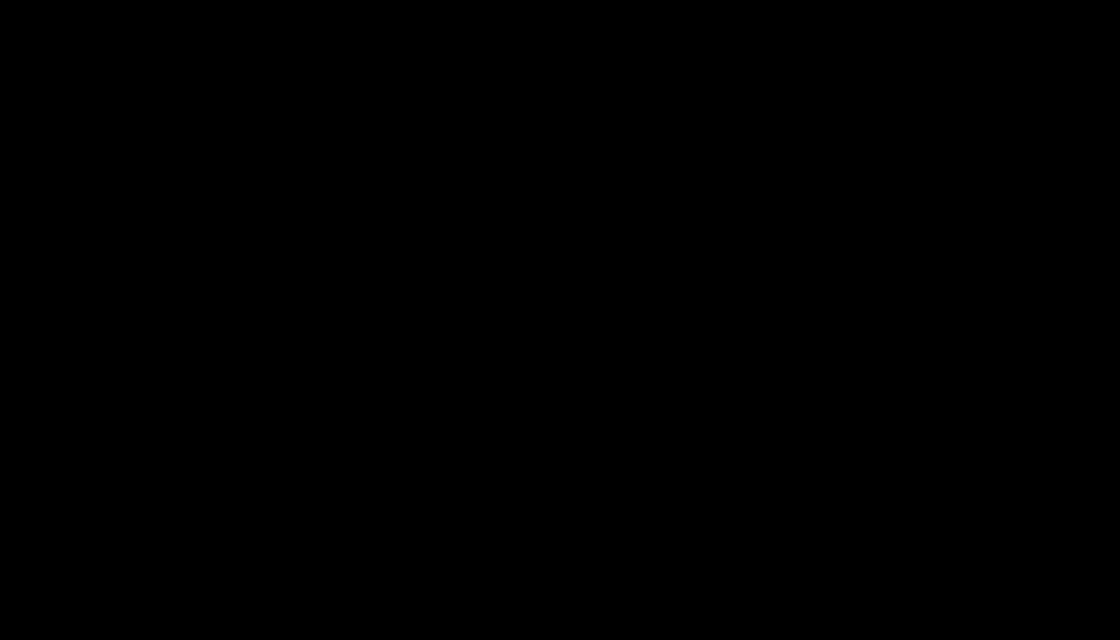 |
| 76 | + |
| 77 | + |
| 78 | +Here's an example of using Figma to React to attach code to a component dragged in from the Team Library: |
| 79 | + |
| 80 | +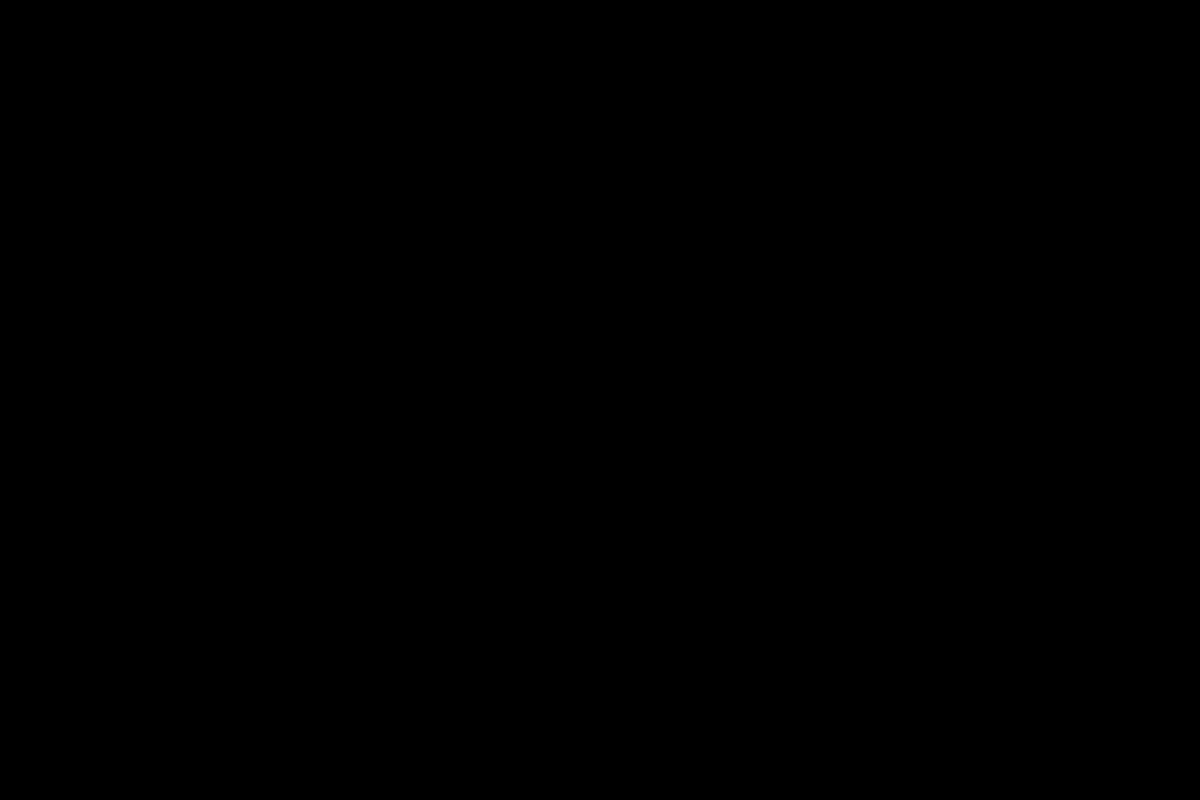 |
| 81 | + |
0 commit comments