Commit c1c2edc
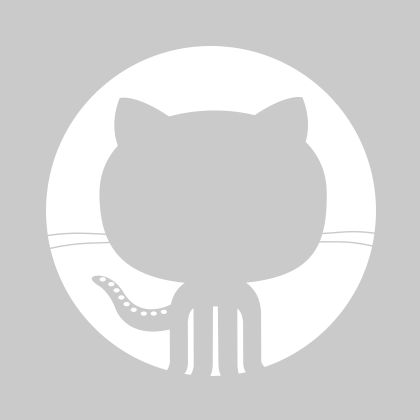
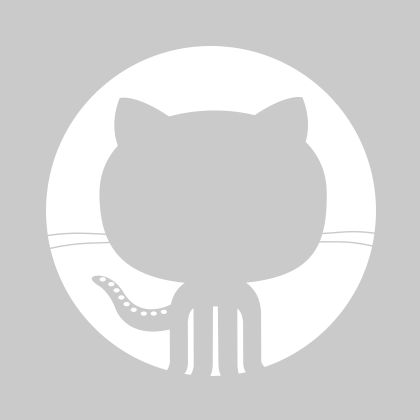
Alexandra Iordache
Samuel Ortiz
1 parent c69c23a commit c1c2edc
File tree
13 files changed
+73
-45
lines changed- src/loader
- aarch64
- x86_64
- bzimage
- elf
13 files changed
+73
-45
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
| 2 | + | |
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
| 2 | + | |
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + |
+16-22
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
12 |
| - | |
| 12 | + | |
13 | 13 |
| |
14 | 14 |
| |
15 | 15 |
| |
| |||
33 | 33 |
| |
34 | 34 |
| |
35 | 35 |
| |
36 |
| - | |
37 |
| - | |
38 |
| - | |
39 |
| - | |
40 |
| - | |
41 |
| - | |
42 |
| - | |
43 |
| - | |
44 |
| - | |
45 |
| - | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
46 | 40 |
| |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
| 41 | + | |
| 42 | + | |
| 43 | + | |
| 44 | + | |
51 | 45 |
| |
52 | 46 |
| |
53 | 47 |
| |
54 | 48 |
| |
55 | 49 |
| |
56 | 50 |
| |
57 |
| - | |
| 51 | + | |
58 | 52 |
| |
59 | 53 |
| |
60 | 54 |
| |
61 |
| - | |
| 55 | + | |
62 | 56 |
| |
63 | 57 |
| |
64 | 58 |
| |
| |||
96 | 90 |
| |
97 | 91 |
| |
98 | 92 |
| |
99 |
| - | |
100 |
| - | |
| 93 | + | |
| 94 | + | |
101 | 95 |
| |
102 | 96 |
| |
103 | 97 |
| |
104 | 98 |
| |
105 | 99 |
| |
106 |
| - | |
107 |
| - | |
| 100 | + | |
| 101 | + | |
108 | 102 |
| |
109 | 103 |
| |
110 | 104 |
| |
| |||
114 | 108 |
| |
115 | 109 |
| |
116 | 110 |
| |
117 |
| - | |
| 111 | + | |
118 | 112 |
| |
119 | 113 |
| |
120 | 114 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
22 | 24 |
| |
23 | 25 |
| |
24 | 26 |
| |
| |||
85 | 87 |
| |
86 | 88 |
| |
87 | 89 |
| |
88 |
| - | |
| 90 | + | |
89 | 91 |
| |
90 | 92 |
| |
91 | 93 |
| |
92 | 94 |
| |
93 |
| - | |
| 95 | + | |
94 | 96 |
| |
95 | 97 |
| |
96 | 98 |
| |
|
File renamed without changes.
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
| 21 | + | |
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
| |||
151 | 151 |
| |
152 | 152 |
| |
153 | 153 |
| |
154 |
| - | |
| 154 | + | |
155 | 155 |
| |
156 | 156 |
| |
157 | 157 |
| |
158 | 158 |
| |
159 |
| - | |
| 159 | + | |
160 | 160 |
| |
161 | 161 |
| |
162 | 162 |
| |
| |||
182 | 182 |
| |
183 | 183 |
| |
184 | 184 |
| |
185 |
| - | |
| 185 | + | |
186 | 186 |
| |
187 | 187 |
| |
188 | 188 |
| |
| |||
252 | 252 |
| |
253 | 253 |
| |
254 | 254 |
| |
255 |
| - | |
256 |
| - | |
| 255 | + | |
| 256 | + | |
257 | 257 |
| |
258 | 258 |
| |
259 | 259 |
| |
260 | 260 |
| |
261 |
| - | |
| 261 | + | |
262 | 262 |
| |
263 | 263 |
| |
264 | 264 |
| |
265 | 265 |
| |
266 |
| - | |
267 |
| - | |
| 266 | + | |
| 267 | + | |
268 | 268 |
| |
269 | 269 |
| |
270 | 270 |
| |
| |||
274 | 274 |
| |
275 | 275 |
| |
276 | 276 |
| |
277 |
| - | |
278 |
| - | |
| 277 | + | |
| 278 | + | |
279 | 279 |
| |
280 | 280 |
| |
281 | 281 |
| |
282 |
| - | |
| 282 | + | |
283 | 283 |
| |
284 | 284 |
| |
285 | 285 |
| |
| |||
290 | 290 |
| |
291 | 291 |
| |
292 | 292 |
| |
293 |
| - | |
| 293 | + | |
| 294 | + | |
294 | 295 |
| |
| 296 | + | |
295 | 297 |
| |
296 |
| - | |
297 |
| - | |
| 298 | + | |
| 299 | + | |
298 | 300 |
| |
299 | 301 |
| |
300 | 302 |
| |
301 | 303 |
| |
302 | 304 |
| |
303 | 305 |
| |
304 |
| - | |
305 |
| - | |
| 306 | + | |
| 307 | + | |
306 | 308 |
| |
307 |
| - | |
| 309 | + | |
308 | 310 |
| |
309 | 311 |
| |
310 | 312 |
| |
|
File renamed without changes.
File renamed without changes.
File renamed without changes.
File renamed without changes.
File renamed without changes.
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + | |
| 18 | + |
0 commit comments