|
1 | 1 | # react-code-view
|
2 | 2 |
|
3 |
| -English | [中文版][readm-cn] |
4 |
| - |
5 |
| -## Why use this component? |
6 |
| - |
7 |
| -Let the code in Markdown run in real time, why is there such a need? |
8 |
| - |
9 |
| -In our front-end team, technical-related documents are written in Markdown. There are often a lot of sample code in the documentation. We hope that when you read the documentation, you can run the sample code to see the rendering interface. |
10 |
| - |
11 |
| -## What are the requirements? |
12 |
| - |
13 |
| -- Let the code in Markdown run and preview. |
14 |
| -- The code can be edited online. |
15 |
| -- Does not affect the layout of the entire document stream. |
16 |
| -- Support React, support code highlighting. |
17 |
| -- Babel can be configured. |
18 |
| - |
19 |
| -After understanding the requirements, I wrote a React component to satisfy these functions, [`react-code-view`](https://github.com/simonguo/react-code-view) , first look at the preview: |
| 3 | +Let the React code in Markdown be rendered to the page, and support online editing. |
20 | 4 |
|
21 | 5 | 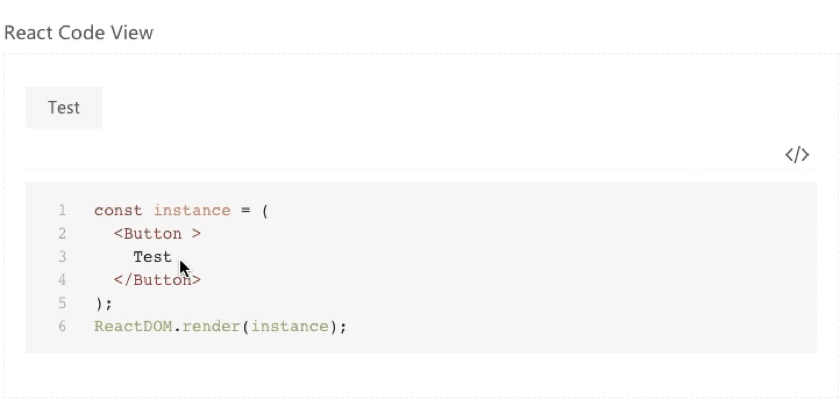
|
22 | 6 |
|
23 |
| -Preview: https://simonguo.github.io/react-code-view/ |
24 |
| - |
25 |
| -## Principle of implementation |
26 |
| - |
27 |
| -- Parse the Markdown document with `markdown-loader` and `html-loader`. |
28 |
| -- Take the code out of the regular expression and give it to `codemirror` |
29 |
| -- Compile the code in `codemirror` through babel and render it to the specified container via `ReactDOM.render` . |
30 |
| - |
31 | 7 | ## Install
|
32 | 8 |
|
33 | 9 | ```
|
34 | 10 | npm install react-code-view
|
35 | 11 | ```
|
36 | 12 |
|
37 |
| -### Configure webpack . |
38 |
| - |
39 |
| -Support for `markdown-loader` needs to be added in webpack. Need to install: |
40 |
| - |
41 |
| -``` |
42 |
| -npm install html-loader --save-dev |
43 |
| -npm install [email protected] --save-dev |
44 |
| -npm install [email protected] --save-dev |
45 |
| -``` |
46 |
| - |
47 |
| -`webpack.config.js` |
| 13 | +### Configure Webpack |
48 | 14 |
|
49 | 15 | ```js
|
| 16 | +// webpack.config.js |
| 17 | +export default { |
| 18 | + module: { |
| 19 | + rules: [ |
| 20 | + { |
| 21 | + test: /\.md$/, |
| 22 | + loader: 'react-code-view/webpack-md-loader' |
| 23 | + } |
| 24 | + ] |
| 25 | + } |
| 26 | +}; |
| 27 | +``` |
50 | 28 |
|
51 |
| -const markdownRenderer = require('react-markdown-reader').renderer; |
| 29 | +#### Options |
52 | 30 |
|
53 |
| -... |
| 31 | +```json |
54 | 32 | {
|
55 |
| - test: /\.md$/, |
56 |
| - use: [{ |
57 |
| - loader: 'html-loader' |
58 |
| - }, { |
59 |
| - loader: 'markdown-loader', |
60 |
| - options: { |
61 |
| - renderer: markdownRenderer(/**languages:Array<string>**/) |
62 |
| - } |
63 |
| - }] |
| 33 | + "parseLanguages": [ |
| 34 | + // Supported languages for highlight.js |
| 35 | + // default: "javascript", "bash", "xml", "css", "markdown", "less", "typescript" |
| 36 | + // See https://github.com/highlightjs/highlight.js/blob/main/SUPPORTED_LANGUAGES.md |
| 37 | + ], |
| 38 | + "htmlOptions": { |
| 39 | + // HTML Loader options |
| 40 | + // See https://github.com/webpack-contrib/html-loader#options |
| 41 | + }, |
| 42 | + "markedOptions": { |
| 43 | + // Pass options to marked |
| 44 | + // See https://marked.js.org/using_advanced#options |
| 45 | + } |
64 | 46 | }
|
65 | 47 | ```
|
66 | 48 |
|
67 |
| -languages 默认值:`["javascript", "bash", "xml", "css", "markdown", "less"]`; |
68 |
| - |
69 |
| -### Add Babel |
| 49 | +**webpack.config.js** |
70 | 50 |
|
71 |
| -The sample code usually uses `ES2015+`, `React`, etc., and needs to compile the code in real time, so introduce `Babel` in `HTML`: |
| 51 | +```js |
72 | 52 |
|
73 |
| -```html |
74 |
| -<script |
75 |
| - type="text/javascript" |
76 |
| - src="//cdn.staticfile.org/babel-standalone/6.24.0/babel.min.js" |
77 |
| -></script> |
| 53 | +export default { |
| 54 | + module: { |
| 55 | + rules: [ |
| 56 | + { |
| 57 | + test: /\.md$/, |
| 58 | + use:[ |
| 59 | + loader: 'react-code-view/webpack-md-loader', |
| 60 | + options:{ |
| 61 | + parseLanguages: ['typescript','rust'] |
| 62 | + } |
| 63 | + ] |
| 64 | + } |
| 65 | + ] |
| 66 | + } |
| 67 | +}; |
78 | 68 | ```
|
79 | 69 |
|
80 |
| -> If `cdn.staticfile.org` is not well accessed in your area, you can change other CDN. |
81 |
| -
|
82 |
| -## Example |
| 70 | +## Usage |
83 | 71 |
|
84 | 72 | ```js
|
85 | 73 | import CodeView from 'react-code-view';
|
86 |
| -import 'react-code-view/lib/less/index.less'; |
87 |
| - |
88 | 74 | import { Button } from 'rsuite';
|
89 | 75 |
|
90 |
| -<CodeView dependencies={{ Button }}>{require('./example.md')}</CodeView>; |
| 76 | +import 'react-code-view/styles/react-code-view.css'; |
| 77 | + |
| 78 | +return ( |
| 79 | + <CodeView |
| 80 | + dependencies={{ |
| 81 | + Button |
| 82 | + }} |
| 83 | + > |
| 84 | + {require('./example.md')} |
| 85 | + </CodeView> |
| 86 | +); |
91 | 87 | ```
|
92 | 88 |
|
93 |
| -The source code is uniformly written in markdown, reference: |
94 |
| -[example.md](https://raw.githubusercontent.com/simonguo/react-code-view/master/docs/example.md) |
95 |
| - |
96 |
| -> The important thing to note here is that the code that needs to be run must be placed in `<!--start-code-->` and `<!--end-code-->` between. |
97 |
| -
|
98 |
| -## API |
| 89 | +The source code is written in markdown, refer to [example.md](https://raw.githubusercontent.com/simonguo/react-code-view/master/docs/example.md) |
99 | 90 |
|
100 |
| -| Name | Type | Default value | Description | |
101 |
| -| --------------------- | -------- | ------------------------------------------- | ------------------------------------------------- | |
102 |
| -| babelTransformOptions | Object | { presets: ['stage-0', 'react', 'es2015'] } | Babel configuration parameters [options][babeljs] | |
103 |
| -| dependencies | Object | | Dependent components. | |
104 |
| -| renderToolbar | Function | | Custom toolbar. | |
105 |
| -| showCode | boolean | true | Display code. | |
106 |
| -| theme | string | 'light' | Theme, options `light` and `dark`. | |
| 91 | +> Note: The code to be rendered must be placed between `<!--start-code-->` and `<!--end-code-->` |
107 | 92 |
|
108 |
| -## Who is using? |
| 93 | +## Props |
109 | 94 |
|
110 |
| -- [React Suite](https://rsuitejs.com/) |
| 95 | +### `<CodeView>` |
111 | 96 |
|
112 |
| -[babeljs]: https://babeljs.io/docs/usage/api/#options |
113 |
| -[readm-cn]: https://github.com/simonguo/react-code-view/blob/master/README_zh-CN.md |
| 97 | +| Name | Type | Default value | Description | |
| 98 | +| -------------- | --------------------------------- | ----------------------- | ------------------------------------------------------------------------- | |
| 99 | +| afterCompile | (code: string) => string | | Executed after compiling the code | |
| 100 | +| beforeCompile | (code: string) => string | | Executed before compiling the code | |
| 101 | +| children | any | | The code to be rendered is executed. Usually imported via markdown-loader | |
| 102 | +| compiler | (code: string) => string | | A compiler that transforms the code. Use swc.transformSync by default | |
| 103 | +| dependencies | object | | Dependent objects required by the executed code | |
| 104 | +| editable | boolean | false | Renders a code editor that can modify the source code | |
| 105 | +| editor | object | | Editor properties | |
| 106 | +| onChange | (code?: string) => void | | Callback triggered after code change | |
| 107 | +| renderToolbar | (buttons: ReactNode) => ReactNode | | Customize the rendering toolbar | |
| 108 | +| sourceCode | string | | The code to be rendered is executed | |
| 109 | +| theme | 'light' , 'dark' | 'light' | Code editor theme, applied to CodeMirror | |
| 110 | +| compileOptions | object | defaultTransformOptions | swc configuration https://swc.rs/docs/configuration/compilation | |
0 commit comments