forked from stepharr/talks
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
2 changed files
with
270 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,140 @@ | ||
# Becoming a CI Cook with Git Hooks pt. 1 | ||
--- | ||
### Git Hooks are scripts that run automatically every time a particular event occurs in a Git repository. | ||
|
||
They are useful for encouraging a commit policy, altering the project environment depending on the state of the repository, and implementing continuous integration. | ||
|
||
|
||
## Setting up git hooks | ||
1. Initialize the repo | ||
```bash | ||
git init | ||
``` | ||
2. Locate the scripts inside the `.git/hooks` directory of a particular repo | ||
3. Ensure the script has the correct permissions to run | ||
``` | ||
chmod +x <script> | ||
``` | ||
|
||
## Git hooks are either client-side or server-side | ||
### Client | ||
- pre-commit | ||
- prepare-commit-msg | ||
- commit-msg | ||
- post-commit | ||
- post-checkout | ||
- pre-rebase | ||
|
||
### Server | ||
- pre-receive | ||
- update | ||
- post-receive | ||
|
||
**Client-side** hook scripts ensure developers follow standards and automate moving code that meet quality standards for deployments/integration. **Server-side** hooks enforce policies to adhere to quality. | ||
## Git hooks and continuous integration | ||
Ensuring that code meets quality is just as important as writing it. You can utilize git hooks to ensure code shared in a team is verified through automated builds and testing, allowing teams to detect problems early. | ||
|
||
Let's look at two local-side git hooks for example. | ||
### Pre-commit hook | ||
- run automated tests | ||
- ensure unnecessary whitespace is removed | ||
- remind developers to include helpful commit messages | ||
#### Example: JS validation before committing | ||
Let's create a pre-commit hook that validates javascript files through [JSLint](www.JSLint.com). We first need to start by downloading the JSLint package from NPM. | ||
``` | ||
npm install -g jslint | ||
``` | ||
Next lets create a pre-commit file in the hooks directory | ||
``` | ||
nano .git/hooks/pre-commit | ||
``` | ||
Now we will add in a script to run .js files through JSLint | ||
|
||
```bash | ||
#!/bin/sh | ||
|
||
# put all js filenames into a variable, if none then exit the hook without an error | ||
files=$(git diff --cached --name-only --diff-filter=ACM | grep ".js$") | ||
if [ "$files" = "" ]; then | ||
exit 0 | ||
fi | ||
|
||
pass=true | ||
|
||
echo "\nValidating JavaScript:\n" | ||
|
||
# run JSlint for each js file, printing if the file passed or failed | ||
for file in ${files}; do | ||
result=$(jslint ${file} | grep "${file} is OK") | ||
if [ "$result" != "" ]; then | ||
echo "\t\033[32mJSLint Passed: ${file}\033[0m" | ||
else | ||
echo "\t\033[31mJSLint Failed: ${file}\033[0m" | ||
pass=false | ||
fi | ||
done | ||
|
||
echo "\nJavaScript validation complete\n" | ||
|
||
# if a file did not pass the linter, let the user know and exit with an error preventing a commit, otherwise allow the commit to proceed | ||
if ! $pass; then | ||
echo "\033[41mCOMMIT FAILED:\033[0m Your commit contains files that should pass JSLint but do not. Please fix the JSLint errors and try again.\n" | ||
exit 1 | ||
else | ||
echo "\033[42mCOMMIT SUCCEEDED\033[0m\n" | ||
fi | ||
``` | ||
Lastly we're going to change the permissions of the file. | ||
|
||
```bash | ||
chmod +x .git/hooks/pre-commit | ||
``` | ||
When you commit the JS files that are staged for the commmit will run through the JSlinter for validation. If they pass standards, you code will then be committed. | ||
|
||
### Post-commit hook | ||
|
||
- deploying changes | ||
- notify of changes | ||
|
||
|
||
#### Example: Deploying changes with a post-commit hook | ||
Create a post-commit file in the hooks directory | ||
``` | ||
nano .git/hooks/post-commit | ||
``` | ||
Add in a script to commit changed files to web server (don't forget to change the `/Path/to/repo/.git` variable). | ||
|
||
```bash | ||
#push automatically to WebServer | ||
#!/bin/bash | ||
unset GIT_INDEX_FILE | ||
sudo git --work-tree=/Library/WebServer/Documents --git-dir=/Path/to/repo/.git checkout -f | ||
``` | ||
Change the permissions of the file | ||
``` | ||
chmod +x .git/hooks/post-commit | ||
``` | ||
Now I'll add a change to my index.html file and commit it | ||
```bash | ||
echo "<p>Here is a change.</p>" >> index.html | ||
git add . | ||
git commit -m "First change" | ||
``` | ||
|
||
After the commit, the post-commit script will utilize my local web server folder to deploy my changes to, allowing me to immediately test my changes after a commit. | ||
## Why is this important? | ||
Automation can remove human errors from your workflow. Here are just a few ways you can utilize git hooks and the respective hook name. | ||
|
||
|
||
- ACLs & testing [**pre-commit**] | ||
- Faster deployments [**post-commit**] | ||
- more helpful commit messages [**prepare-commit-msg**] | ||
- ensure branch is ready for commits [**post-checkout**] | ||
- prevent feature branches that are already merged to 'dev' branch from getting rebased [**pre-rebase**] | ||
- reject code that doesn't adhere to the teams standards [**pre-receive**] | ||
- enable push to deploy for faster releases [**post-receive**] | ||
|
||
Git hooks can also be source controlled through git and creating a symbolic link to the file in .git/hooks. For example: | ||
```bash | ||
ln -s /path/to/repo/pre-commit-jslint /path/to/repo/.git/hooks/pre-commit | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,130 @@ | ||
<!-- background: #fff --> | ||
<!-- color: #000 --> | ||
<!-- font: frutiger --> | ||
|
||
# "Overkilling" Your Git Repo with Gitflow | ||
by Stephon Harris | ||
|
||
<script> | ||
Hi! I'm Stephon out of the DC Office and today I'm going to tell you abut managing your source-controlled workflow with Gitflow. | ||
</script> | ||
*** | ||
# Bad workflows need to die | ||
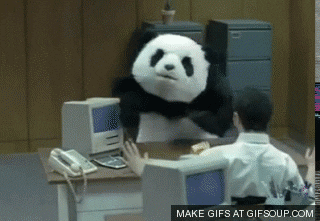 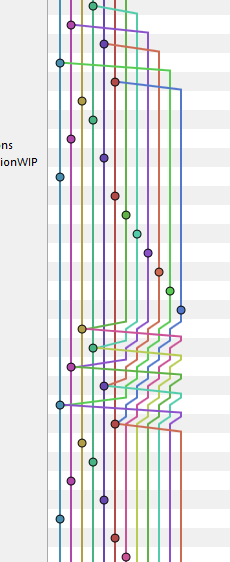 | ||
You need a branching strategy to give your projects life | ||
|
||
<script> | ||
Managing the flow of code can grow scary using Git when you have a plethora of branches and convoluted merges. Gitflow is a branching model that is collaborative, scalable, and sustainable. | ||
</script> | ||
*** | ||
# Wait, But Why? | ||
- Organize code for development and production-ready stages | ||
- Provide workflow for integrating changes | ||
- Parallel development of features | ||
- Continuously deliver through releases and hotfixes | ||
<script> | ||
Gitflow wrangles up commits and merges with a clear and formal workflow for promoting production-ready code, and separating the development of new features, while still allowing for breakfixes to be made. | ||
</script> | ||
*** | ||
# OK, How? | ||
 | ||
<script> | ||
There's a simple methodology behind this madness. Just study this image and you've got it! Just kidding, I'll walk you through how each of these branches are used. | ||
</script> | ||
*** | ||
# So there are Main Branches | ||
 | ||
 | ||
- Exist for the life of the project | ||
- **Develop** contains work in progress | ||
- **Master** contains production releases | ||
<script> | ||
Develop and Master are the two main branches. The Develop branch is for the code you're working to release. The Master branch is for the code you have released. These branches will exist for the life of the project. You don't ever commit directly to these branches, but rather merge in code. | ||
</script> | ||
*** | ||
# ...and Developmental Branches | ||
 | ||
 | ||
 | ||
- Exist until merged into develop or release branches, or abandoned | ||
- **Feature** contains developmental new features | ||
- **Release** contains prepared releases from **Develop** | ||
- **Hotfix** contains production fixes | ||
<script> | ||
The three branches you actually do work and commit to are feature branches, release branches, and hot-fixes. Feature branches are for developing as many new features as you'd like. Feature branches promote code to the Develop branch. Release branches, are for when you're ready to promote code from the Develop branch to Master for production. Making changes on the release branch is only for minor tweaks and release notes. And then there are hot-fixes that are for squashing any bugs and making minor changes that need to go directly to production code, and - most importantly - are not a new feature. When code is promoted to Develop and/or Master, the commits are integrated into the main branches and then the developmental branch is deleted. If you decide to abandon any changes before merging them into one of the main branches you can delete that developmental branch and continue to develop without having to back out of any changes. | ||
</script> | ||
*** | ||
# What? | ||
\*<\sigh>\* | ||
To put it plain and simple: | ||
<script> | ||
I know that can be a lot to take in so let me break it down for you. | ||
</script> | ||
*** | ||
# Start a feature -> Do some work -> Finish feature = Develop has new feature | ||
 | ||
<script> | ||
Look at it this way. These gold circles represent commits and the grey circles represent merges. You start this flow by creating a feature, then working on it, and finally integrating that feature back into the develop branch or abandoning it. | ||
</script> | ||
*** | ||
# Create a release -> Tweak and write release note -> Finish Release = Production and Develop has new release | ||
 | ||
<script> | ||
When you're code is solid and you're ready to release it to the world, you create a release branch, add in minor changes and any release notes, and then it's merged into the develop and master branches. | ||
<script> | ||
*** | ||
# Create a hotfix -> Correct some code -> Finish hotfix = Production and Develop have hotfix | ||
 | ||
<script> | ||
If there are any breakfixes that need to be made, you start a hotfix, make the code changes and then it's merged into the main branches. | ||
</script> | ||
*** | ||
# Repeat. | ||
<script> | ||
And this beautiful cycle is repeated for eternity, or at least for the life of your project. | ||
</script> | ||
*** | ||
# Demo | ||
## Gitflow_init - Source Tree | ||
1. Initialize & First commit | ||
2. Create Feature | ||
## Gitflow CLI | ||
git flow init | ||
git flow feature start SolarSystem | ||
git flow feature finish SolarSystem | ||
## Gitflow_feature - Source Tree | ||
3. Create a release | ||
4. Demonstrate how a release is merged into master and develop | ||
5. Create a hot-fix | ||
<script> | ||
I'd like to now show you git flow in action | ||
</script> | ||
*** | ||
# Keep in Mind | ||
- Developmental branches are given a respective naming convention (e.g. **feature**/Neptune, **release**/10000000, **hotfix**/pluto) | ||
- Anything that goes to production is tagged (e.g. v1.0) | ||
- Rebase when finishing **Feature** to keep **Develop** commit history linear and clean | ||
- You can use [Git Hooks](https://github.com/jaspernbrouwer/git-flow-hooks) to automate naming tags/versions | ||
<script> | ||
Some things you may want to remember. There is a naming convention associated with branches and as you create new features, releases and hotfixes, they will take on their respective prefix. Production-level code in master is tagged. After finishing a feature, I suggest rebasing to keep your code pristine. Once you've found your gitflow groove, you can even automate tag numbers being bumped and specifying tag messages through git hook scripts. | ||
</script> | ||
*** | ||
# Woah! | ||
 | ||
*You're welcome!* | ||
<script> | ||
Yea, Gitflow is pretty awesome and it makes your code play nice with others and more importantly helps you and your team release code quicker and cleaner, just how coding should be. Any questions? | ||
</script> | ||
*** | ||
# References | ||
<script> | ||
Attached is a reference to more material on Gitflow and how to set it up on your computer for a CLI and SourceTree. | ||
</script> | ||
- Gitflow's Branching Model Strategy- http://nvie.com/posts/a-successful-git-branching-model/ | ||
- Gitflow SourceTree- https://blog.sourcetreeapp.com/2012/08/01/smart-branching-with-sourcetree-and-git-flow/ | ||
- Gitflow CLI- https://github.com/nvie/gitflow | ||
- Continuous Delivery & Gitflow- https://www.atlassian.com/continuous-delivery/continuous-delivery-workflows-with-feature-branching-and-gitflow | ||
- Git Hooks- https://github.com/jaspernbrouwer/git-flow-hooks | ||
- Glitter Toss- http://i.giphy.com/xTiTnEHBh7qapyuvwQ.gif |